Ajax获取首页数据–axios
- 项目安装axios
分析:首页由5个组件组成,每一个组件都有自己的数据,那么每个组件都发送一个ajax请求。如果这样做首页就会发送5个ajax请求,网站的性能会很低。
解决:Home.vue组件中发送ajax是最好的选择,这个组件获取ajax请求的数据传给每个子组件中,只用请求一次。
- 引入axios
1
| import axios from "axios";
|
- 使用
1 2 3 4 5 6 7 8 9 10
| methods: { getHomeInfo() { axios.get("/api/index.json").then(this.getHomeInfoSucc); } }, mounted() { this.getHomeInfo(); }
|
这里我们的使用的是本地模拟的数据json文件,开发环境中使用转发机制
vue中提供了一个代理功能
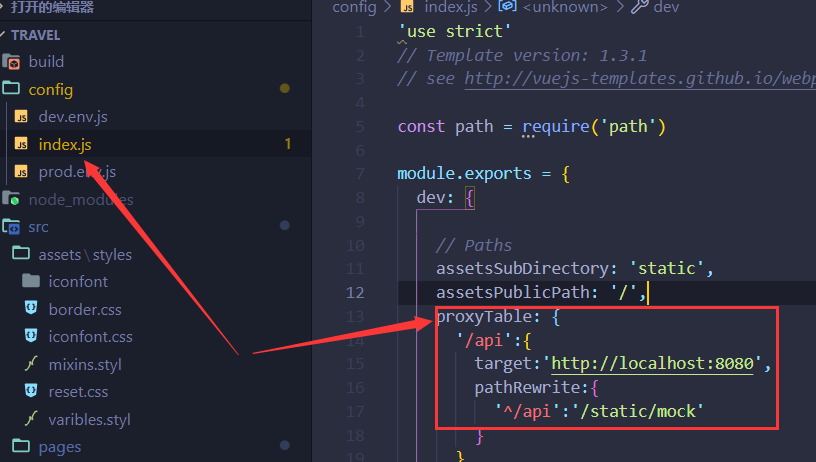
index.json
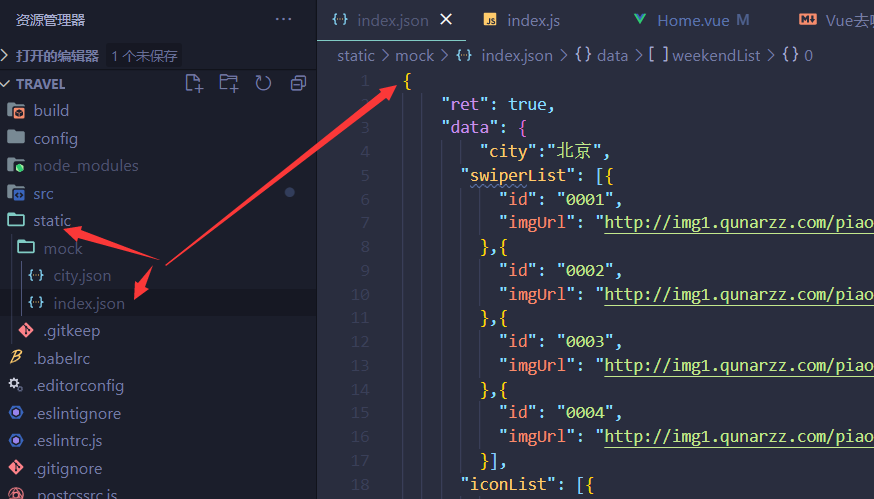
首页父子组件数据传递
Home.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60
| <template> <div> <home-header :city="city"></home-header> <home-swiper :list="swiperList"></home-swiper> <home-icons :list="iconList"></home-icons> <home-recommend :list="recommendList"></home-recommend> <home-weekend :list="weekendList"></home-weekend> </div> </template>
<script> import HomeHeader from "./components/Header"; import HomeSwiper from "./components/Swiper"; import HomeIcons from "./components/Icons"; import HomeRecommend from "./components/Recommend"; import HomeWeekend from "./components/Weekend"; import axios from "axios"; export default { name: "Home", components: { HomeHeader, HomeSwiper, HomeIcons, HomeRecommend, HomeWeekend }, data() { return { city: "", swiperList: [], iconList: [], recommendList: [], weekendList: [] }; }, methods: { getHomeInfo() { axios.get("/api/index.json").then(this.getHomeInfoSucc); }, getHomeInfoSucc(res) { res = res.data; if (res.ret && res.data) { const data = res.data; this.city = res.data.city; this.swiperList = data.swiperList; this.iconList = data.iconList; this.recommendList = data.recommendList; this.weekendList = data.weekendList; } } }, mounted() { this.getHomeInfo(); } }; </script>
<style></style>
|
父组件给子组件传值
使用props,父组件可以使用props向子组件传递数据。
- Swiper.vue中小问题
默认显示的是最后一页的图片
分析:当页面还没接收数据的时候,也就是还没接收ajax获取的Array数组时,这时候接收的是外面获取的空数组。
解决
1
| <swiper :options="swiperOptions" v-if="showSwiper">
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| <script> export default { name: "HomeSwiper", props: { list: Array }, data() { return { swiperOptions: { pagination: ".swiper-pagination", loop: true } }; }, computed: { showSwiper() { return this.list.length; } } }; </script>
|